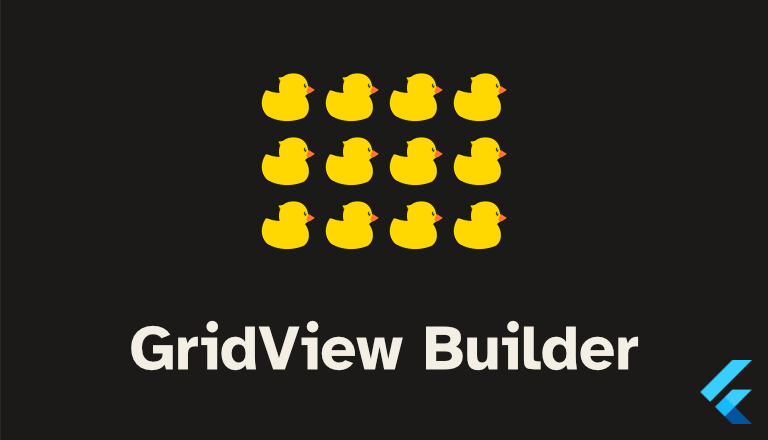
Rendering grids in Flutter using GridView builder
Mar 12, 2024
In this article, we’re going to talk about displaying items in a grid.
Check out a complete example on GitHub: https://github.com/minibuildsio/flutter_grid_example.
Create a grid with GridView.builder()
The function GridView.builder()
provides a convenient way to build a grid of widgets. The main arguments of the builder are the following:
GridView.builder(
gridDelegate: ...,
itemCount: ...,
itemBuilder: ...
)
gridDelegate
: The defines the layout of the grid e.g. number of columns or tile size and spacing. There are two implementations:SliverGridDelegateWithFixedCrossAxisCount
: fixed number of columnsSliverGridDelegateWithMaxCrossAxisExtent
: the number of columns increases when a maximum tile width is reached
itemCount
: The number of items in the grid, this can be omitted to build an infinite grid. Typically the length of a list containing the items.itemBuilder
: A function that creates a widget at a particular position. TheitemBuilder
is only called when a tile comes into view so the number of grid tiles can be large without causing any performance issues.
Ducky grid example
The example below creates a grid that is 3 tiles wide where each tile is an image of the minibuilds duck.
class DuckyGrid extends StatelessWidget {
final int duckyCount;
const DuckyGrid(this.duckyCount, {super.key});
@override
Widget build(BuildContext context) {
return GridView.builder(
gridDelegate: const SliverGridDelegateWithFixedCrossAxisCount(
crossAxisCount: 3,
mainAxisSpacing: 16.0,
crossAxisSpacing: 16.0,
),
itemCount: duckyCount,
itemBuilder: (context, index) => Container(
color: Colors.black87,
child: Column(
children: [
Image.asset('assets/minibuilds-ducky.png'),
Text(
'Duck ${index + 1}',
style: const TextStyle(color: Colors.white),
)
],
),
),
);
}
}