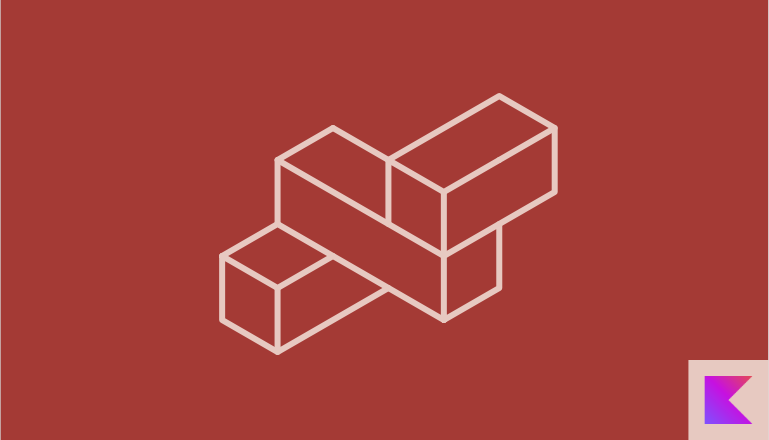
Writing more readable code using Kotlin's extension methods
Kotlin extension functions are a powerful feature that allows you to add new functions to existing classes without modifying their source code. For example, adding an isEven()
function to Int
. You can extend any class even classes that are otherwise unmodifiable such as String
as well as generic classes such as List<Float>
.
The basic syntax of an extension function is similar to a regular function with the addition of the class you wish to extend before the function name i.e.
fun Class.functionName(parameters): ReturnType {
// function body
}
For example, adding isEven()
to an Int
would look like.
fun Int.isEven(): Boolean {
return this % 2 == 0
}
// or more succinctly
fun Int.isEven() = this % 2 == 0
Now the isEven()
function will be available on any Int
, and can be used like so.
5.isEven() // == false
Extension functions can help you improve the readability of your code by making the intent very clear. For example, the following line of code, which removes all odd numbers from a list, is pretty easy to understand.
val evens = numbers.filter { it.isEven() }
When compared to the following.
val evens = numbers.filter { it % 2 == 0 }