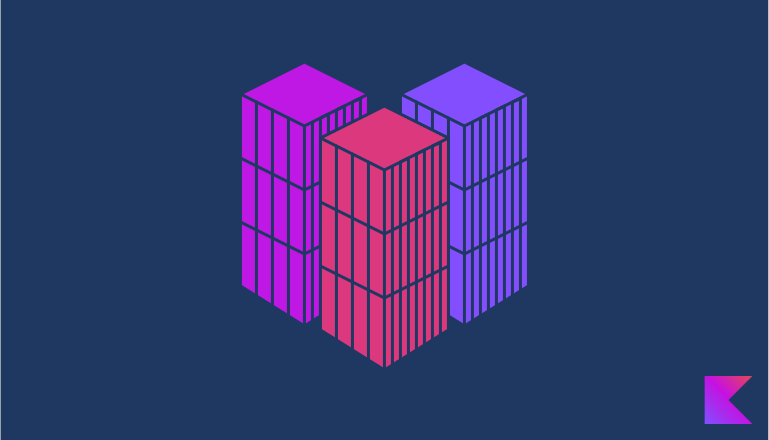
Using partition and groupby to split lists in Kotlin
Dec 15, 2023
In this article, we’ll talk about some options Kotlin provides for splitting a list into parts based on a function.
partition
: split a list into two using a predicate the first list containing items where the predicate is true and the second containing the rest.groupBy
: split a list into a map using a function to determine the key.
Splitting into Two Lists using partition { ... }
partition
allows us to split a list by a predicate. The example below splits a list of numbers into two based on this parity of the numbers. partition
returns a Pair
that can be conveniently decomposed into selected and the test e.g. (evens, odds)
.
val numbers = 1..10
val (evens, odds) = numbers.partition { it.isEven() }
// evens = [2, 4, 6, 8, 10]
// odds = [1, 3, 5, 7, 9]
Where isEven
is an extension method:
fun Int.isEven(): Boolean {
return this % 2 == 0
}
Splitting into a Map using groupBy { ... }
groupBy
allows us to split a list into a map where the key is determined by a function. The example below splits a list of names into map grouping by the length of the names.
val names = listOf("Sally", "Ben", "Todd", "Josh", "Jade")
val namesByLength = names.groupBy { it.length }
// namesByLength = {5=[Sally], 3=[Ben], 4=[Todd, Josh, Jade]}