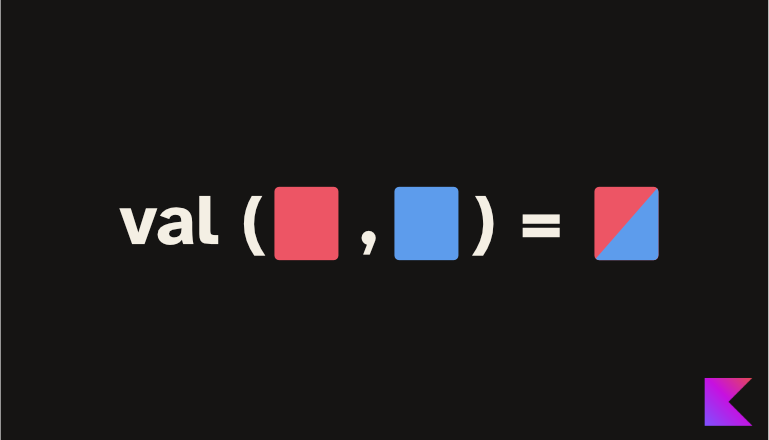
Kotlin Syntax Destructuring Declaration
Occasionally, we want to create a set of variables from the fields of an object for example the name
, age
, and height
fields from a person
object. Kotlin provides the destructuring declaration syntax to achieve this like so.
val (name, age, height) = person
// equivalent to
val name = person.component1() // = person.name
val age = person.component2() // = person.age
val height = person.component3() // = person.height
Where person
is an instance of the class Person
below.
data class Person(val name: String, val age: Int, val height: Int, val weight: Int)
The order of the variables must match the order of the fields in the constructor. So if you wish to ‘miss’ a field you’d have to do something like val (name, age, _, weight) = person
.
Pair and Triple Destructuring Declaration
Kotlin provides the classes Pair and Triple to represent a generic pair or triple of two or three values. These objects can be destructured in the same way as any other object i.e.
val (first, second) = functionThatReturnsAPair()
Pair destructuring is useful when using functions such as partition
which splits a list in two depending on whether the items meet some condition. partition
returns a Pair of the items that matched and the rest.
val numbers = 1..10
val (evens, odds) = numbers.partition { it.isEven() }
I personally really like the above example as the intent of what is being achieved is crystal clear. Certainly when compared to the Java equivalent below which forces you to Map<Boolean, List<Integer>>
and then pick out the values.
Map<Boolean, List<Integer>> groups = numbers.stream()
.collect(Collectors.partitioningBy(s -> s % 2 == 0));
List<Integer> evens = groups.get(true);
List<Integer> odds = groups.get(false);
The Java version also has a potential bug in it as the numbers
list might not contain an even or odd number resulting in groups.get(...)
returning null
.